domain driven design pdf
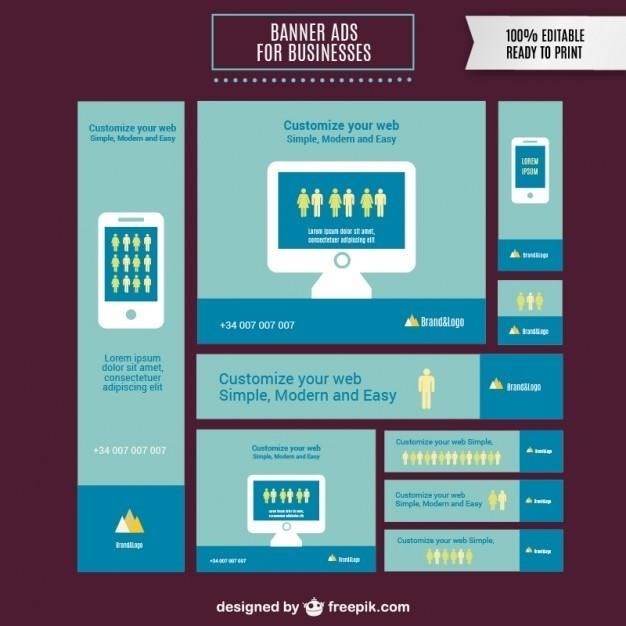
Domain-Driven Design (DDD)⁚ A Comprehensive Guide
Domain-Driven Design (DDD) is a software development approach that emphasizes the importance of understanding the domain of a system and using that understanding to guide the design and development process. DDD focuses on creating a rich domain model that encapsulates the business logic and rules of the domain. This approach helps to ensure that the software is aligned with the needs of the business and that it can be easily adapted to changing requirements. DDD is a powerful approach that can help to build complex and maintainable software systems. This guide will provide you with a comprehensive overview of DDD‚ including its key concepts‚ benefits‚ and implementation strategies.
Introduction to Domain-Driven Design
Domain-Driven Design (DDD) is a software development approach that prioritizes understanding the core domain of a system and utilizing that knowledge to guide the design and development process. It focuses on creating a robust domain model that embodies the intricate business logic and rules of the domain‚ effectively bridging the gap between business reality and the code. DDD’s emphasis on a deep understanding of the domain sets it apart from traditional software development approaches that often prioritize technical concerns over business requirements.
The core principle of DDD is to create a model that accurately reflects the domain‚ allowing developers to build software systems that are aligned with the needs of the business. This approach involves close collaboration between developers and domain experts‚ ensuring that the software accurately captures the complexities and nuances of the business domain. By fostering a shared understanding of the domain‚ DDD promotes communication and collaboration among team members‚ leading to more effective software development.
The concept of DDD was introduced by Eric Evans in his seminal book‚ “Domain-Driven Design⁚ Tackling Complexity in the Heart of Software.” Evans’s work revolutionized software development by highlighting the importance of understanding the business domain and incorporating that understanding into the design and development process. DDD has since become a widely accepted approach to building complex software systems‚ particularly in organizations that require software to accurately reflect the intricacies of their business operations.
Benefits of Domain-Driven Design
Domain-Driven Design (DDD) offers numerous benefits that contribute to the development of high-quality‚ maintainable‚ and adaptable software systems. DDD’s focus on understanding and modeling the business domain leads to software that accurately reflects the complexities of the real world‚ resulting in several key advantages⁚
Improved Communication⁚ DDD promotes a shared understanding of the domain among developers and domain experts‚ fostering clear communication and collaboration. This shared language‚ known as the “Ubiquitous Language‚” reduces ambiguity and ensures that everyone is on the same page‚ leading to more efficient and effective development.
Enhanced Code Maintainability⁚ The use of a rich domain model in DDD makes the codebase more understandable and maintainable. By encapsulating business logic within the domain model‚ developers can easily understand the purpose and functionality of different components. This clarity makes it easier to modify and extend the software without introducing unintended consequences.
Increased Adaptability⁚ DDD enables software systems to adapt more readily to changing business requirements. By focusing on the core domain‚ DDD allows developers to easily modify and extend the system’s functionality without affecting other parts of the codebase. This agility is crucial in today’s rapidly evolving business environment.
Reduced Complexity⁚ DDD helps to manage complexity by breaking down large‚ complex systems into smaller‚ more manageable units. By focusing on specific aspects of the domain‚ DDD makes it easier to understand and reason about the system‚ leading to more efficient development and maintenance.
Improved Business Alignment⁚ DDD ensures that the software system aligns with the business’s needs and goals. By focusing on the domain‚ DDD ensures that the software system accurately reflects the business processes and rules‚ resulting in a system that is more valuable to the organization.
Key Concepts in Domain-Driven Design
Domain-Driven Design (DDD) revolves around a set of fundamental concepts that guide the design and development of software systems. These concepts‚ often referred to as “building blocks” of DDD‚ provide a framework for understanding and modeling the business domain effectively. Here are some key concepts in DDD⁚
Bounded Contexts⁚ DDD emphasizes the importance of recognizing different contexts within a system‚ each with its own unique set of rules and language. Bounded contexts are distinct parts of the system with clear boundaries‚ allowing for independent development and evolution. This approach prevents conflicting interpretations of the domain and ensures that the software accurately reflects the complexities of the real world.
Ubiquitous Language⁚ DDD stresses the importance of a shared language‚ known as the “Ubiquitous Language‚” between developers‚ domain experts‚ and stakeholders. This common vocabulary ensures that everyone involved in the project understands the domain concepts and terminology in the same way. The Ubiquitous Language promotes effective communication‚ reduces ambiguity‚ and ensures that the software accurately reflects the business’s needs.
Domain Model⁚ The core of DDD is the domain model‚ which represents the business logic and rules of the system. The domain model encapsulates the key concepts‚ relationships‚ and behavior of the domain. This model serves as a bridge between the business world and the software system‚ ensuring that the software accurately reflects the business’s needs and can adapt to changing requirements.
Aggregates⁚ Aggregates are clusters of related objects that represent a cohesive unit within the domain model. They ensure data consistency and integrity by controlling access to their constituent objects‚ promoting a consistent and predictable behavior within the domain model.
Value Objects⁚ Value objects are immutable objects that represent data without an identity. They are primarily defined by their attributes and are used to model concepts that are defined by their values‚ such as money‚ dates‚ or addresses. Value objects contribute to a more concise and expressive domain model.
Strategic Design Patterns in DDD
Strategic design patterns in Domain-Driven Design (DDD) focus on the high-level architecture and organization of a software system. They address how to divide a complex domain into manageable parts and how to manage the interactions between those parts. These patterns are crucial for building scalable‚ maintainable‚ and adaptable software systems. Here are some key strategic design patterns in DDD⁚
Bounded Contexts⁚ Bounded contexts are a fundamental strategic pattern in DDD. They represent distinct parts of a system‚ each with its own unique language‚ rules‚ and model. This pattern helps to manage complexity by breaking down a large and intricate domain into smaller‚ more manageable units. Bounded contexts enable independent development and evolution‚ preventing conflicts and ensuring that each context reflects its specific business needs.
Context Map⁚ The Context Map is a visual representation of the relationships between different bounded contexts in a system. It shows how these contexts interact‚ including collaborations‚ shared language‚ and dependencies. The Context Map is a valuable tool for understanding the overall architecture of a system and for managing the relationships between different teams working on different parts of the system.
Anti-Corruption Layer⁚ The Anti-Corruption Layer acts as a buffer between a bounded context and external systems‚ protecting the integrity of the domain model. It translates the language and data formats of external systems to the domain model’s language‚ ensuring consistency and preventing corruption. This pattern is essential for integrating with legacy systems or external services without compromising the domain model’s integrity.
Shared Kernel⁚ In some cases‚ different bounded contexts may share a common set of rules and models. The Shared Kernel pattern allows these contexts to share a common set of code‚ ensuring consistency and reducing duplication. However‚ it’s crucial to maintain a clear separation of concerns and avoid tight coupling between the contexts.
Customer/Supplier: The Customer/Supplier pattern defines a relationship between two bounded contexts where one context (the customer) relies on the services provided by another context (the supplier). This pattern promotes loose coupling and allows for independent development and evolution of the contexts.
Conformist⁚ The Conformist pattern describes a situation where one bounded context adapts its language and model to conform to another context. This pattern is useful when integrating with external systems that cannot be easily changed. It helps to maintain consistency and reduce integration complexity.
Bounded Contexts
Bounded Contexts are a fundamental strategic pattern in Domain-Driven Design (DDD) that plays a crucial role in managing complexity within large and intricate software systems. The concept is based on the idea of dividing a system into distinct parts‚ each with its own unique language‚ rules‚ and model. This approach allows for independent development and evolution of these parts‚ preventing conflicts and ensuring that each context reflects its specific business needs.
Think of a large organization with multiple departments‚ each with its own set of processes‚ rules‚ and terminology. A bounded context would represent one of these departments‚ such as “Sales‚” “Inventory‚” or “Customer Service.” Each department has its own way of understanding and interacting with the business domain‚ and it’s important to reflect this in the software system.
By defining bounded contexts‚ you create a clear separation of concerns and prevent the spread of complexity. This approach promotes modularity and maintainability‚ making it easier to understand and modify the system. Each context can have its own language‚ model‚ and implementation‚ allowing for greater flexibility and adaptability.
Here are some key benefits of using Bounded Contexts in DDD⁚
- Reduced Complexity⁚ Breaking down a complex domain into smaller‚ more manageable units reduces the cognitive load on developers‚ making the system easier to understand and work with.
- Independent Development⁚ Teams can work on different bounded contexts in parallel‚ speeding up development and reducing the risk of conflicts.
- Flexibility and Adaptability⁚ Each context can evolve independently‚ allowing for changes in the business domain without impacting other parts of the system.
- Improved Communication⁚ By defining a clear language and model for each context‚ you improve communication between developers and domain experts.
Bounded Contexts are a powerful tool for managing complexity in large software systems. By understanding and applying this pattern‚ you can create software systems that are more maintainable‚ adaptable‚ and aligned with the needs of the business domain.
Ubiquitous Language
Ubiquitous Language‚ a cornerstone concept in Domain-Driven Design (DDD)‚ is a shared vocabulary that bridges the communication gap between developers and domain experts. It acts as a common language that everyone involved in the software development process uses to understand and discuss the business domain. This shared language ensures that everyone is on the same page‚ reducing misunderstandings and fostering collaboration.
Imagine a scenario where developers are building a system for an online retail store. They might use terms like “product‚” “order‚” and “customer” without fully understanding the nuances of these concepts from a business perspective. This can lead to inconsistencies and errors in the software.
Ubiquitous Language addresses this challenge by creating a common vocabulary that captures the specific terminology and nuances of the business domain. It involves working closely with domain experts to identify and define terms that accurately represent the business processes‚ entities‚ and relationships.
This shared language is not just a list of words; it’s a living document that evolves over time as the understanding of the domain deepens. Developers use this language to design the software‚ write code‚ and document the system. Domain experts use it to communicate their requirements and validate the software’s functionality.
The key benefits of Ubiquitous Language include⁚
- Improved Communication⁚ A shared language removes ambiguity and ensures that everyone involved in the project understands the domain in the same way.
- Reduced Errors⁚ By using a consistent language‚ developers are less likely to make mistakes when designing and implementing the software.
- Enhanced Collaboration⁚ Developers and domain experts can work together more effectively when they share a common understanding of the business domain.
- Increased Agility⁚ A well-defined Ubiquitous Language makes it easier to adapt the software to changing requirements.
By adopting a Ubiquitous Language‚ you create a foundation for successful domain-driven design‚ ensuring that your software accurately reflects the needs of the business domain and fosters effective communication between all stakeholders.
Tactical Design Patterns in DDD
Tactical design patterns in Domain-Driven Design (DDD) provide practical‚ low-level blueprints for structuring code and modeling domain concepts within the context of a bounded context. These patterns help ensure that the domain model is cohesive‚ maintainable‚ and aligned with the ubiquitous language. They are essential for implementing the strategic design patterns and achieving the overall goals of DDD.
Tactical design patterns focus on specific aspects of the domain model‚ such as⁚
- Encapsulation⁚ Concealing internal details and exposing only the necessary interfaces for interaction.
- Data Integrity⁚ Ensuring the consistency and validity of data through constraints and rules.
- Behavior⁚ Defining how objects interact and respond to events.
- Relationships⁚ Modeling the connections and dependencies between domain objects.
Some commonly used tactical design patterns in DDD include⁚
- Entities⁚ Objects with identity and state‚ representing core domain concepts. They typically have a lifecycle and can change over time.
- Value Objects⁚ Objects that represent a specific value and are immutable. They are often used to represent data types like addresses‚ phone numbers‚ or currency.
- Aggregates⁚ Clusters of related entities grouped together to maintain data consistency and enforce business rules. They have a root entity that serves as the entry point for interactions.
- Domain Services⁚ Objects that encapsulate complex business logic that doesn’t belong to any specific entity. They provide a way to perform actions that affect multiple entities.
- Domain Events⁚ Objects that represent occurrences within the domain model. They are used to communicate changes and trigger specific actions.
By applying these tactical design patterns‚ developers can create a domain model that is both robust and expressive‚ accurately representing the complexities of the business domain.